matplotlib.patches.Rectangle#
- class matplotlib.patches.Rectangle(xy, width, height, *, angle=0.0, rotation_point='xy', **kwargs)[source]#
Bases:
Patch
A rectangle defined via an anchor point xy and its width and height.
The rectangle extends from
xy[0]
toxy[0] + width
in x-direction and fromxy[1]
toxy[1] + height
in y-direction.: +------------------+ : | | : height | : | | : (xy)---- width -----+
One may picture xy as the bottom left corner, but which corner xy is actually depends on the direction of the axis and the sign of width and height; e.g. xy would be the bottom right corner if the x-axis was inverted or if width was negative.
- Parameters:
- xy(float, float)
The anchor point.
- widthfloat
Rectangle width.
- heightfloat
Rectangle height.
- anglefloat, default: 0
Rotation in degrees anti-clockwise about the rotation point.
- rotation_point{'xy', 'center', (number, number)}, default: 'xy'
If
'xy'
, rotate around the anchor point. If'center'
rotate around the center. If 2-tuple of number, rotate around this coordinate.
- Other Parameters:
- **kwargs
Patch
properties Property
Description
a filter function, which takes a (m, n, 3) float array and a dpi value, and returns a (m, n, 3) array and two offsets from the bottom left corner of the image
unknown
bool
antialiased
or aabool or None
CapStyle
or {'butt', 'projecting', 'round'}BboxBase
or Nonebool
Patch or (Path, Transform) or None
edgecolor
or eccolor or None
facecolor
or fccolor or None
bool
str
{'/', '\', '|', '-', '+', 'x', 'o', 'O', '.', '*'}
bool
JoinStyle
or {'miter', 'round', 'bevel'}object
linestyle
or ls{'-', '--', '-.', ':', '', (offset, on-off-seq), ...}
linewidth
or lwfloat or None
bool
list of
AbstractPathEffect
None or bool or float or callable
bool
(scale: float, length: float, randomness: float)
bool or None
str
bool
float
- **kwargs
- get_patch_transform()[source]#
Return the
Transform
instance mapping patch coordinates to data coordinates.For example, one may define a patch of a circle which represents a radius of 5 by providing coordinates for a unit circle, and a transform which scales the coordinates (the patch coordinate) by 5.
- property rotation_point#
The rotation point of the patch.
- set(*, agg_filter=<UNSET>, alpha=<UNSET>, angle=<UNSET>, animated=<UNSET>, antialiased=<UNSET>, bounds=<UNSET>, capstyle=<UNSET>, clip_box=<UNSET>, clip_on=<UNSET>, clip_path=<UNSET>, color=<UNSET>, edgecolor=<UNSET>, facecolor=<UNSET>, fill=<UNSET>, gid=<UNSET>, hatch=<UNSET>, height=<UNSET>, in_layout=<UNSET>, joinstyle=<UNSET>, label=<UNSET>, linestyle=<UNSET>, linewidth=<UNSET>, mouseover=<UNSET>, path_effects=<UNSET>, picker=<UNSET>, rasterized=<UNSET>, sketch_params=<UNSET>, snap=<UNSET>, transform=<UNSET>, url=<UNSET>, visible=<UNSET>, width=<UNSET>, x=<UNSET>, xy=<UNSET>, y=<UNSET>, zorder=<UNSET>)[source]#
Set multiple properties at once.
Supported properties are
Property
Description
a filter function, which takes a (m, n, 3) float array and a dpi value, and returns a (m, n, 3) array and two offsets from the bottom left corner of the image
scalar or None
unknown
bool
antialiased
or aabool or None
(left, bottom, width, height)
CapStyle
or {'butt', 'projecting', 'round'}BboxBase
or Nonebool
Patch or (Path, Transform) or None
edgecolor
or eccolor or None
facecolor
or fccolor or None
bool
str
{'/', '\', '|', '-', '+', 'x', 'o', 'O', '.', '*'}
unknown
bool
JoinStyle
or {'miter', 'round', 'bevel'}object
linestyle
or ls{'-', '--', '-.', ':', '', (offset, on-off-seq), ...}
linewidth
or lwfloat or None
bool
list of
AbstractPathEffect
None or bool or float or callable
bool
(scale: float, length: float, randomness: float)
bool or None
str
bool
unknown
unknown
(float, float)
unknown
float
- set_angle(angle)[source]#
Set the rotation angle in degrees.
The rotation is performed anti-clockwise around xy.
- set_bounds(*args)[source]#
Set the bounds of the rectangle as left, bottom, width, height.
The values may be passed as separate parameters or as a tuple:
set_bounds(left, bottom, width, height) set_bounds((left, bottom, width, height))
- set_xy(xy)[source]#
Set the left and bottom coordinates of the rectangle.
- Parameters:
- xy(float, float)
- property xy#
Return the left and bottom coords of the rectangle as a tuple.
Examples using matplotlib.patches.Rectangle
#
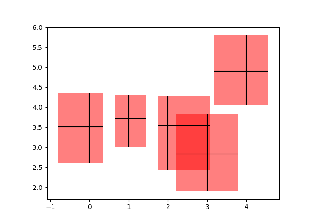
Creating boxes from error bars using PatchCollection